Progressively Enhancing Vault Security
Identity Vault offers various configuration options for securing a Vault, including VaultType
and DeviceSecurityType
. These options allow developers to dynamically select the most appropriate Vault configuration based on the end user's device capabilities.
In this tutorial, we'll explore how developers can take advantage of Identity Vault's Device
API to enhance security progressively based on the user's device capabilities.
Before starting, take a look at the workflow below. We will be programming this workflow.
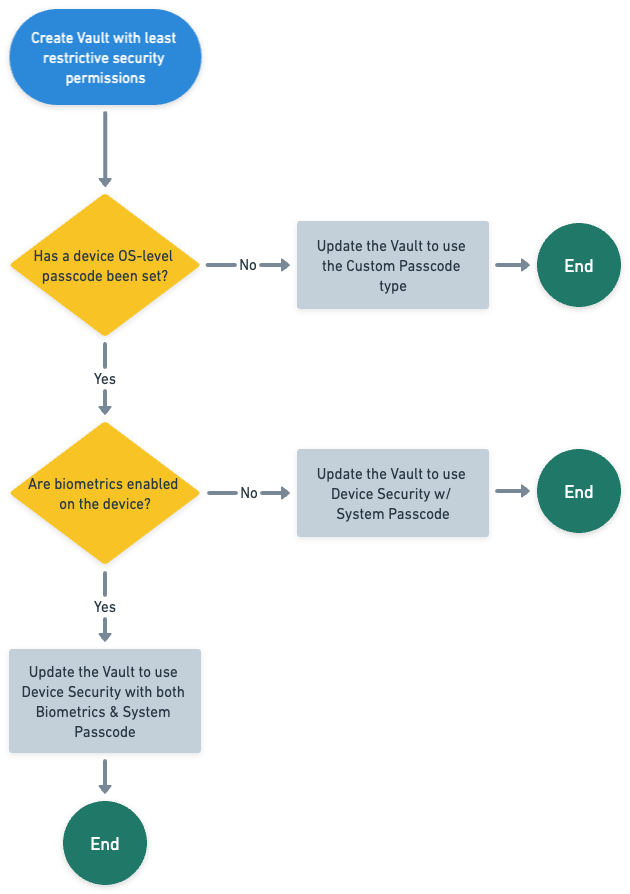
Create an initialization factory
_10import { isPlatform } from '@ionic/core';_10import { BrowserVault, IdentityVaultConfig, Vault } from '@ionic-enterprise/identity-vault';_10_10const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_10 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_10};
Start by creating a factory function that builds either the actual Vault, if we are on a device, or a browser-based "Vault" suitable for development in the browser.
This is a standard step Ionic recommends taking as you develop any project with Identity Vault, so your development workflow is unimpeded.
Initialize the Vault
Start with the least restrictive security options
The least restrictive security option a Vault can have is the SecureStorage
vault type mode.
This configuration does not require the device to have any security hardware to store data within a Vault.
_22import { isPlatform } from '@ionic/core';_22import { BrowserVault, IdentityVaultConfig, Vault, VaultType, DeviceSecurityType } from '@ionic-enterprise/identity-vault';_22_22const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_22 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_22};_22_22const initialOptions: IdentityVaultConfig = {_22 key: 'io.ionic.progressiveSecurityVault',_22 type: VaultType.SecureStorage,_22 deviceSecurityType: DeviceSecurityType.None,_22 lockAfterBackgrounded: 2000,_22 shouldClearVaultAfterTooManyFailedAttempts: true,_22 customPasscodeInvalidUnlockAttempts: 2,_22 unlockVaultOnLoad: false_22};_22_22const createProgressivelyEnhancedVault = async () => {_22 const vault = createVault(initialOptions);_22 return vault;_22};_22export createProgressivelyEnhancedVault;
Progressively enhance security
Check for device security hardware
_28import { isPlatform } from '@ionic/core';_28import { BrowserVault, IdentityVaultConfig, Vault, VaultType, DeviceSecurityType, Device } from '@ionic-enterprise/identity-vault';_28_28const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_28 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_28};_28_28const initialOptions: IdentityVaultConfig = {_28 key: 'io.ionic.progressiveSecurityVault',_28 type: VaultType.SecureStorage,_28 deviceSecurityType: DeviceSecurityType.None,_28 lockAfterBackgrounded: 2000,_28 shouldClearVaultAfterTooManyFailedAttempts: true,_28 customPasscodeInvalidUnlockAttempts: 2,_28 unlockVaultOnLoad: false_28};_28_28const createProgressivelyEnhancedVault = async () => {_28 const vault = createVault(initialOptions);_28 if (!(await Device.isSystemPasscodeSet())) {_28 await vault.updateConfig({ _28 ...vault.config, _28 type: VaultType.CustomPasscode_28 });_28 }_28 return vault;_28};_28export default createProgressivelyEnhancedVault;
You can test if a device has security hardware enabled by checking if a device passcode has been set.
If not, we can add a layer of security to the Vault by using the CustomPasscode
vault type. This will require a user to set a custom passcode that will be used to access the Vault.
Note: Learn more about
CustomPasscode
in this cookbook.
Check for biometric hardware
_34import { isPlatform } from '@ionic/core';_34import { BrowserVault, IdentityVaultConfig, Vault, VaultType, DeviceSecurityType, Device } from '@ionic-enterprise/identity-vault';_34_34const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_34 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_34};_34_34const initialOptions: IdentityVaultConfig = {_34 key: 'io.ionic.progressiveSecurityVault',_34 type: VaultType.SecureStorage,_34 deviceSecurityType: DeviceSecurityType.None,_34 lockAfterBackgrounded: 2000,_34 shouldClearVaultAfterTooManyFailedAttempts: true,_34 customPasscodeInvalidUnlockAttempts: 2,_34 unlockVaultOnLoad: false_34};_34_34const createProgressivelyEnhancedVault = async () => {_34 const vault = createVault(initialOptions);_34 if (!(await Device.isSystemPasscodeSet())) {_34 await vault.updateConfig({ _34 ...vault.config, _34 type: VaultType.CustomPasscode_34 });_34 } else if (!(await Device.isBiometricsEnabled())) {_34 await vault.updateConfig({_34 ...vault.config,_34 type: VaultType.DeviceSecurity,_34 deviceSecurityType: DeviceSecurityType.SystemPasscode,_34 });_34 }_34 return vault;_34};_34export default createProgressivelyEnhancedVault;
If the previous check passes, we'll want to check to see if the device has biometrics enabled and enrolled.
In the event the device only has a device passcode enabled, we can still use device level security letting Identity Vault know that this Vault will be secured using it.
_40import { isPlatform } from '@ionic/core';_40import { BrowserVault, IdentityVaultConfig, Vault, VaultType, DeviceSecurityType, Device } from '@ionic-enterprise/identity-vault';_40_40const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_40 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_40};_40_40const initialOptions: IdentityVaultConfig = {_40 key: 'io.ionic.progressiveSecurityVault',_40 type: VaultType.SecureStorage,_40 deviceSecurityType: DeviceSecurityType.None,_40 lockAfterBackgrounded: 2000,_40 shouldClearVaultAfterTooManyFailedAttempts: true,_40 customPasscodeInvalidUnlockAttempts: 2,_40 unlockVaultOnLoad: false_40};_40_40const createProgressivelyEnhancedVault = async () => {_40 const vault = createVault(initialOptions);_40 if (!(await Device.isSystemPasscodeSet())) {_40 await vault.updateConfig({ _40 ...vault.config, _40 type: VaultType.CustomPasscode_40 });_40 } else if (!(await Device.isBiometricsEnabled())) {_40 await vault.updateConfig({_40 ...vault.config,_40 type: VaultType.DeviceSecurity,_40 deviceSecurityType: DeviceSecurityType.SystemPasscode,_40 });_40 } else {_40 await vault.updateConfig({_40 ...vault.config,_40 type: VaultType.DeviceSecurity,_40 deviceSecurityType: DeviceSecurityType.SystemPasscode,_40 });_40 }_40 return vault;_40};_40export default createProgressivelyEnhancedVault;
Secure the Vault using biometric security
If both checks pass the device can use biometrics to lock and unlock the Vault.
Identity Vault has the ability to lock/unlock using the device passcode, biometrics, or both.
Note: Take a look at the DeviceSecurityType enumeration for more information.
Create an initialization factory
Start by creating a factory function that builds either the actual Vault, if we are on a device, or a browser-based "Vault" suitable for development in the browser.
This is a standard step Ionic recommends taking as you develop any project with Identity Vault, so your development workflow is unimpeded.
Initialize the Vault
Start with the least restrictive security options
The least restrictive security option a Vault can have is the SecureStorage
vault type mode.
This configuration does not require the device to have any security hardware to store data within a Vault.
Progressively enhance security
Check for device security hardware
You can test if a device has security hardware enabled by checking if a device passcode has been set.
If not, we can add a layer of security to the Vault by using the CustomPasscode
vault type. This will require a user to set a custom passcode that will be used to access the Vault.
Note: Learn more about
CustomPasscode
in this cookbook.
Check for biometric hardware
If the previous check passes, we'll want to check to see if the device has biometrics enabled and enrolled.
In the event the device only has a device passcode enabled, we can still use device level security letting Identity Vault know that this Vault will be secured using it.
Secure the Vault using biometric security
If both checks pass the device can use biometrics to lock and unlock the Vault.
Identity Vault has the ability to lock/unlock using the device passcode, biometrics, or both.
Note: Take a look at the DeviceSecurityType enumeration for more information.
_10import { isPlatform } from '@ionic/core';_10import { BrowserVault, IdentityVaultConfig, Vault } from '@ionic-enterprise/identity-vault';_10_10const createVault = (config: IdentityVaultConfig): Vault | BrowserVault => {_10 return isPlatform("hybrid") ? new Vault(config) : new BrowserVault(config);_10};